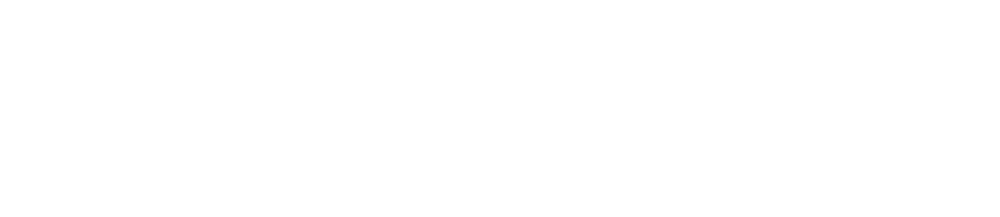
Endpoint address:
https://rpc.btcs.com
Builder+ by BTCS is our newly developed Ethereum block builder which utilizes advanced algorithms to meticulously construct optimized blocks for on-chain validation designed to maximize validator earnings.
(Builder+ services are not accessible from or provided to the following countries: Cuba, Iran, North Korea, Russia, Syria, and Venezuela)
eth_sendBundle
{
"jsonrpc": "2.0",
"id": 1,
"method": "eth_sendBundle",
"params": [
{
txs, // Array[String], A list of signed transactions to execute in an atomic bundle, list can be empty for bundle cancellations
blockNumber, // (Optional) String, a hex-encoded block number for which this bundle is valid. Default, current block number
minTimestamp, // (Optional) Number, the minimum timestamp for which this bundle is valid, in seconds since the unix epoch
maxTimestamp, // (Optional) Number, the maximum timestamp for which this bundle is valid, in seconds since the unix epoch
revertingTxHashes, // (Optional) Array[String], A list of tx hashes that are allowed to revert or be discarded
replacementUuid, // (Optional) String, any arbitrary string that can be used to replace or cancel this bundle
}
]
}
Important: If the refundPercent field is set, the builder will construct a refund transaction automatically. However, if the refund amount does not cover the cost of the transaction (i.e., gas_used * base_fee), the bundle will be discarded.
Refund Example
Consider the following Bundle:
-
- TXN 1 – User swap (Base fee: 50 Gwei, Piority fee 3 Gwei, Gas: 280k)
- TXN 2 – Backrun (Base fee: 50 Gwei, Priority fee 100 Gwei, Gas: 150k)
- refundPercent: 90%
- Current block base fee: 50 Gwei
Calculation:
- ETH reward of the last transaction in the bundle = (150k x 100 Gwei) = 15,000 Gwei
- ETH reward after transfer transaction fees = 15000 – (21k x 50 Gwei) = 13,950 Gwei
- Refund amount = 0.9 x 13,950 Gwei = 12,555 Gwei
CURL example
curl -s --data '{"jsonrpc": "2.0","id": "1","method": "eth_sendBundle","params": [{"txs": ["0x12…ab","0x34..cd"], "blockNumber": "0x102286B","replacementUuid": "abcd1234"}]}' -H "Content-Type: application/json" -X POST https://rpc.btcs.com
Response example
{"result":{"bundleHash":"0x164d7d41f24b7f333af3b4a70b690cf93f636227165ea2b699fbb7eed09c46c7"},"error":null,"id":1}
Bundle Hash
Here is the algorithm for determining the bundle hash:
// Bundle hash implementation.
// Different to standard as we include Block Number and Replacement UUID.
func (b *BundleArgs) Hash() common.Hash {
bundleHasher := sha3.NewLegacyKeccak256()
bundleHasher.Write([]byte(b.BlockNumber))
bundleHasher.Write([]byte(b.ReplacementUuid))
for _, tx := range b.Txs {
bundleHasher.Write(tx.Hash)
}
bundleHash := common.BytesToHash(bundleHasher.Sum(nil))
return bundleHash
}
eth_sendRawTransaction
Also applies to eth_sendPrivateRawTransaction
{
“jsonrpc”: “2.0”,
“id”: “1”,
“method”: “eth_sendRawTransaction”,
“params”: [“0x…4b”], // Signed raw tx hex
}
CURL example
curl -s –data ‘{“jsonrpc”: “2.0”,”id”: “1”,”method”: “eth_sendRawTransaction”,”params”: [“0x…12”]}’ -H “Content-Type: application/json” -X POST https://builder.btcs.com
Response example
{“result”:200,”error”:null,”id”:1}
eth_sendPrivateTransaction
Also applies to eth_sendPrivateRawTransaction
{
“jsonrpc”: “2.0”,
“id”: “1”,
“method”: “eth_sendPrivateTransaction”,
“params”: [
{
tx, // Signed raw tx hex
maxBlockNumber, // (Optional) String, a hex-encoded string representing the block number of the last block in which the transaction should be included
}
]
}
CURL example
curl -s –data ‘{“jsonrpc”: “2.0”,”id”: “1”,”method”: “eth_sendPrivateTransaction”,”params”: [{“tx”: “0x12…ab”}]}’ -H “Content-Type: application/json” -X POST https://builder.btcs.com
Response example
{“result”:200,”error”:null,”id”:1}
eth_cancelBundle
{
“jsonrpc”: “2.0”,
“id”: 1,
“method”: “eth_cancelBundle”,
“params”: [
{
replacementUuid, // String, to uniquely identify submission
}
]
}
Important: the replacementUuid must have been set when the bundle was submitted.
CURL example
curl -s –data ‘{“jsonrpc”: “2.0”,”id”: “1”,”method”: “eth_cancelBundle”, “params”: [{“replacementUuid”: “abcd1234”}]}’ -H “Content-Type: application/json” -X POST https://builder.btcs.com
Response example
{“result”:200,”error”:null,”id”:1}
Important: We cannot guarantee that the bundle will be canceled if the cancellation is submitted within 4 seconds of the final relay submission.
Our builder will submit only using the following public keys:
0x8042acb1f21ff5ee9d0035c2ea5d9a09c3810b048ba28f89e9a94e8e76c5fdd5bebce9b88af4e489ccd1daf735b829bb
0xa3ad1da9373ae97c21274629668d5b585addc0dfd1b323f0651159df409603f7b0c1fa90237164f817301b6a5733a220
0xb9810c6839e91b063f8d2dc7acddc60599341600351d7f09d195cbc148307d31b667b52ccfb6559c61a0bdbc06ecdf86
0xb7c52ab445df2be2b6910f7710de2c236a77130b44a69486abfd89e8fcadc3fd6e545fa02329dc9c30a2c23b727ddab9
0xb7c5beefbaea0b050764617a764d571929a8a250e9e1226a3c31c738d05ea1fc8b3f2d455e15d623f6a64d52e4f84761
Any other public key should be considered unrelated to our builder.